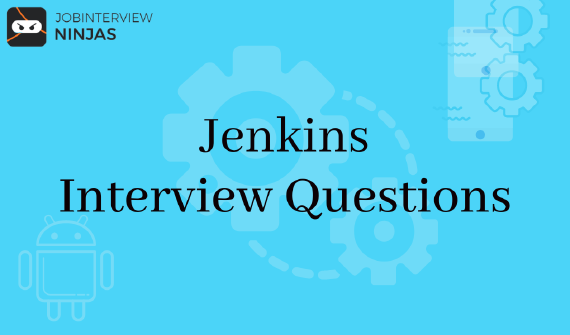
Top 40 Jenkins Interview Questions And Answers For Freshers/Experienced
If you are looking for a career in software development, then Jenkins is definitely worth exploring. This widely used …
PL/SQL is a popular topic to be asked in technical interviews. So for your benefit here we bring you Top 50+ PL/SQL interview questions and answers. Practice these PL/SQL interview questions to crack the interview questions. Here we have tried to cover PL/SQL basic interview questions, PL/SQL technical interview questions, and PL/SQL coding questions which can be asked during the interview.
About PL/SQL: PL/SQL full form is Procedural Language extensions to SQL (Structured Query Language). It was made by Oracle to overcome the issues of SQL and easily build and handling of crucial applications in a comprehensive manner.
3. What is the basic structure of PL/SQL?
4. Tell the use of WHERE CURRENT OF in cursors?
5. How will you assign a name to an unnamed PL/SQL Exception Block?
6. Define Trigger? Name some instances of using “Triggers”.
7. When does a DECLARE block of PL/SQL become mandatory?
8. What are the different comments in a PL/SQL code and how do you write it?
9. Tell the use of the WHEN clause in the trigger?
12. For what database links are used?
13. Write a PL/SQL code to find whether a given string is palindrome or not.
15. Write a PL/SQL program to find the sum of digits of a number.
16. Show some predefined exceptions.
17. How to find an open cursor in a PL SQL Block?
18. Tell any 2 PL/SQL cursor exceptions.
19. What are the available PL SQL developer packages?
20. Explain 3 basic parts of a trigger.
21. What does fetching a cursor do?
22. What does closing a cursor do?
24. Differentiate Anonymous blocks and sub-programs.
25. Differentiate DECODE and CASE.
26. Explain autonomous transactions.
27. Difference between SGA and PGA.
28. Where do you find Pre_defined_functions.
31. Can we execute 2 queries together in a Distributed Database System?
32. Explain Raise_application_error.
33. How do you convert date into Julian date format?
35. What does the PL/SQL package contain?
36. What are the methods to trace the PL/SQL code?
37. What does a hierarchical profiler do?
39. What does the PLV (PL/Vision) package offer?
40. Use of PLVprs and PLVprsps?
41. How to copy a file to file content and file to PL/SQL table?
42. How are exceptions handled in advance PL/SQL?
43. What are the issues that occur while writing log information to a database table?
44. What is the function used to transfer a PL/SQL table log to a database table?
45. Why is PLVtab the easiest to access the PL/SQL table?
46. What does PLVtab enable to show the contents of PL/SQL tables?
47. How can you save messages in a table?
48. Use of the “module procedure” function in PL/SQL?
49. What do PLVcmt and PLVrb do in PL/SQL?
50. Name some character functions?
3. What is the basic structure of PL/SQL?
PL/SQL follows the basic BLOCK structure. Each code contains SQL and PL/SQL statements that together make a PL/SQL block.
Each PL/SQL block has these 3 sections:
[DECLARE]
--declaration statements (optional)
BEGIN
--execution statements
[EXCEPTION]
--exception handling statements (optional)
END;
4. Tell the use of WHERE CURRENT OF in cursors?
This clause represents the current row from an explicit cursor. This clause applies updates and deletes the row which is currently under consideration without mentioning the row ID.
Syntax:
UPDATE table_name SET field=new_value WHERE CURRENT OF cursor_name
5. How will you assign a name to an unnamed PL/SQL Exception Block?
By using Pragma called EXCEPTION_INIT we can name a PL/SQL Exception Block. It allows the programmer to command the compiler to provide custom error messages based on business logic by overtaking the predefined messages during the compilation time. Syntax: DECLARE exception_name EXCEPTION; PRAGMA EXCEPTION_INIT (exception_name, error_code); BEGIN // PL/SQL Logic EXCEPTION WHEN exception_name THEN // Steps to handle exception END;
6. Define Trigger? Name some instances of using “Triggers”.
As the name says, ‘Trigger’ means to start or ‘activate’ something. In PL/SQL, it is a stored procedure that tells what action the database will take when an event related to it is performed.
· Syntax:
TRIGGER trigger_name
trigger_event
[ restrictions ]
BEGIN
actions_of_trigger;
END;
Some instances where Triggers can be used:
7. When does a DECLARE block of PL/SQL become mandatory?
8. What are the different comments in a PL/SQL code and how do you write it?
Sentences that do not have any effect on the functionality and are only used for the core purpose of enhancing the readability are called Comments. They are of two types:
Example:
SET SERVEROUTPUT ON;
DECLARE
-- Hi There! I am a single line comment.
var_name varchar2(40) := ‘I love PL/SQL’ ;
BEGIN
/*
Hi! I am a multi line
comment. I span across
multiple lines
*/
dbms_output.put_line(var_name);
END;
/
Output:
I love PL/SQL
9. Tell the use of the WHEN clause in the trigger?
11. Write a PL/SQL procedure for selecting some records from the database using some parameters as filters.
CREATE PROCEDURE get_employee_details @salary nvarchar(30)
AS
BEGIN
SELECT * FROM ib_employee WHERE salary = @salary;
END;
12. For what database links are used?
13. Write a PL/SQL code to find whether a given string is palindrome or not.
DECLARE -- Declared variables string, letter, reverse_string where string is the original string. string VARCHAR2(10) := 'abccba'; letter VARCHAR2(20); reverse_string VARCHAR2(10); BEGIN FOR i IN REVERSE 1..LENGTH(string) LOOP letter := SUBSTR(string, i, 1); -- concatenate letter to reverse_string variable reverse_string := reverse_string ||''||letter; END LOOP; IF reverse_string = string THEN dbms_output.Put_line(reverse_string||''||' is palindrome'); ELSE dbms_output.Put_line(reverse_string ||'' ||' is not palindrome'); END IF; END;
14. Write a PL/SQL program to convert each digit of a given number into its corresponding word format.
DECLARE -- declare necessary variables -- num represents the given number -- number_to_word represents the word format of the number -- str, len and digit are the intermediate variables used for program execution num INTEGER; number_to_word VARCHAR2(100); digit_str VARCHAR2(100); len INTEGER; digit INTEGER; BEGIN num := 123456; len := LENGTH(num); dbms_output.PUT_LINE('Input: ' ||num); -- Iterate through the number one by one FOR i IN 1..len LOOP digit := SUBSTR(num, i, 1); -- Using DECODE, get the str representation of the digit SELECT Decode(digit, 0, 'Zero ', 1, 'One ', 2, 'Two ', 3, 'Three ', 4, 'Four ', 5, 'Five ', 6, 'Six ', 7, 'Seven ', 8, 'Eight ', 9, 'Nine ') INTO digit_str FROM dual; -- Append the str representation of digit to final result. number_to_word := number_to_word || digit_str; END LOOP; dbms_output.PUT_LINE('Output: ' ||number_to_word); END; Input: 12345 Output: One Two Three Four Five
15. Write a PL/SQL program to find the sum of digits of a number.
DECLARE --Declare variables num, sum_of_digits and remainder of datatype Integer num INTEGER; sum_of_digits INTEGER; remainder INTEGER; BEGIN num := 123456; sum_of_digits := 0; -- Find the sum of digits until original number doesn't become null WHILE num <> 0 LOOP remainder := MOD(num, 10); sum_of_digits := sum_of_digits + remainder; num := TRUNC(num / 10); END LOOP; dbms_output.PUT_LINE('Sum of digits is '|| sum_of_digits); END; Input: 9874 Output: 28
16. Show some predefined exceptions.
DUP_VAL_ON_INDEX
ZERO_DIVIDE
NO_DATA_FOUND
TOO_MANY_ROWS
CURSOR_ALREADY_OPEN
INVALID_NUMBER
INVALID_CURSOR
PROGRAM_ERROR
TIMEOUT _ON_RESOURCE
STORAGE_ERROR
LOGON_DENIED
VALUE_ERROR
etc.
17. How to find an open cursor in a PL SQL Block?
18. Tell any 2 PL/SQL cursor exceptions.
Cursor_Already_Open Invaid_cursor
19. What are the available PL SQL developer packages?
20. Explain 3 basic parts of a trigger.
21. What does fetching a cursor do?
22. What does closing a cursor do?
24. Differentiate Anonymous blocks and sub-programs.
25. Differentiate DECODE and CASE.
DECODE and CASE statements are the same but the difference is CASE is an extended version of DECODE. DECODE won’t allow Decision-making statements in its place.
select decode(totalsal=12000,’high’,10000,’medium’) as decode_tesr from smp where smpno in (10,12,14,16);
This statement returns an error.
CASE is used in PL SQL, but DECODE is used in PL SQL through SQL only.
26. Explain autonomous transactions.
27. Difference between SGA and PGA.
28. Where do you find Pre_defined_functions.
MERGE combines multiple DML statements into one.
Syntax: merge into table name
using(query)
on(join condition)
when not matched then
[insert/update/delete] command
when matched then
[insert/update/delete] command
31. Can we execute 2 queries together in a Distributed Database System?
32. Explain Raise_application_error.
33. How do you convert date into Julian date format?
35. What does the PL/SQL package contain?
36. What are the methods to trace the PL/SQL code?
37. What does a hierarchical profiler do?
39. What does the PLV (PL/Vision) package offer?
40. Use of PLVprs and PLVprsps?
PLVprs: It is an extension and the lowest level of string parsing for PL/SQL functionality
PLVprsps: It is the highest level package of parsing PL/SQL source code into separate atomics. It depends on other parsing packages to get work done.
41. How to copy a file to file content and file to PL/SQL table?
42. How are exceptions handled in advance PL/SQL?
43. What are the issues that occur while writing log information to a database table?
44. What is the function used to transfer a PL/SQL table log to a database table?
45. Why is PLVtab the easiest to access the PL/SQL table?
46. What does PLVtab enable to show the contents of PL/SQL tables?
47. How can you save messages in a table?
There are two ways to save a message in a table:
Use add_text procedure to load individual messages with calls
Use load_from_dbms procedure to load sets of messages from a database table
48. Use of the “module procedure” function in PL/SQL?
49. What do PLVcmt and PLVrb do in PL/SQL?
PLVcmt and PLVrb are the two packages that manage the transaction processes in PL/SQL applications.
PLVcmt: It wraps logic and complexity to manage commit processing
PLVrb: It is a programmatic interface to roll-back activity
50. Name some character functions?
You can cover the above given PL/SQL interview questions and answers to ace your interview. In the above section, we have tried to cover all questions related to the PL/SQL system which was asked in the Interview of freshers and professionals. If you have any questions or need help related to interview questions and answers on PL/SQL then feel free to reach us.
Want to prepare for these languages:
If you are looking for a career in software development, then Jenkins is definitely worth exploring. This widely used …
In this post, we will cover a few Linux interview questions and their answers. So, let’s get started. In this …