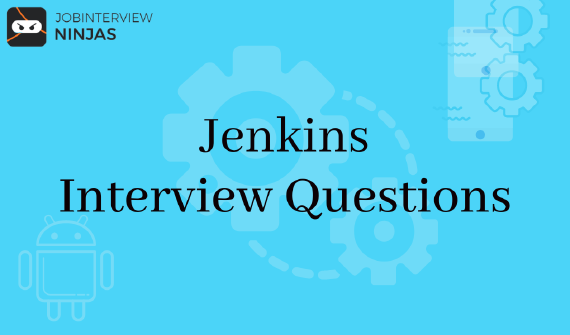
Top 40 Jenkins Interview Questions And Answers For Freshers/Experienced
If you are looking for a career in software development, then Jenkins is definitely worth exploring. This widely used …
Preparing for an upcoming job interview in which Node JS is required then go through this page. It is good to brush up on your knowledge and skills and well prepare for your interview beforehand. Generally, there are a few questions are asked related to Node.js interview questions unless the interview revolves around node.js only. So we recommend you to prepare from Node.js interview questions and answer and focus on your interview.
About Node.js: It is a popularly known server-side platform that is an open-source, back-end JavaScript runtime environment that executes on the V8 engine and runs JavaScript code outside a web browser.
Also, prepare for TypeScript interview questions, Networking interview questions, and other language interview questions from here.
1. Tell steps of “Control Flow” to control the functions calls?
2. Explain some timing features of Node.js?
3. Why do we need to use Node.js?
4. Tell some advantages of using promises than callbacks?
6. Explain Node.js single-threaded?
7. Create a simple server in Node.js that brings back Hello World?
8. Explain different types of API functions of Node.js?
10. Tell two arguments that async.queue takes as input?
11. Explain the core purpose of module.exports?
12. Tools to use consistent code style?
14. If Node.js is single-threaded then how it can handle concurrency?
15. Explain the use of async wait in node.js?
19. Explain Reactor Pattern in Node.js?
20. Explain Event Emitter in Node.js?
21. Explain thread pool and library that handles it in Node.js
22. What is WASI and why is it being introduced?
23. How to calculate the duration of async operations?
24. How to calculate the performance of async operations?
25. Explain callback in Node.js.
27. Where do we use Node.js most frequently?
29. Explain the reasons why Node.js is chosen over other technologies like Java and PHP?
30. Name the database used with Node.js?
31. Name the common libraries used in Node.js?
32. Discuss the command required to import external libraries?
33. Explain the meaning of event-driven programming?
34. Explain Event Loop in Node.js?
35. Explain the Express.js package?
36. Tell us the way to implement async in Node.js?
37. Explain the control flow function?
38. Explain piping in Node.js?
39. Explain buffer class in Node.js?
40. Explain different types of HTTP requests?
41. Discuss first-class function in Javascript?
42. Explain Node.js and its works?
43. Tell us how Node.js overcomes the issue of blocking of I/O operations?
44. Explain the exit codes of Node.js?
1. Tell steps of “Control Flow” to control the functions calls?
2. Explain some timing features of Node.js?
3. Why do we need to use Node.js?
4. Tell some advantages of using promises than callbacks?
6. Explain Node.js single-threaded?
7. Create a simple server in Node.js that brings back Hello World?
var http = require(“http”);
http.createServer(function (request, response) {
response.writeHead(200, {‘Content-Type’: ‘text/plain’});
response.end(‘Hello World\n’);
}).listen(3000);
8. Explain different types of API functions of Node.js?
10. Tell two arguments that async.queue takes as input?
11. Explain the core purpose of module.exports?
module.exports are basically used to reveal functions of a specific module or file to be used somewhere else in the project. This can be used to capture all same functions in a file which further develops the project structure.
For example, let assume you have filed for all utils functions with util to acquire solutions in various programming languages of a problem statement.
const getSolutionInJavaScript = async ({
problem_id
}) => {
…
};
const getSolutionInPython = async ({
problem_id
}) => {
…
};
module.exports = { getSolutionInJavaScript, getSolutionInPython }
Thus using module.exports you are able to use these functions in some other file:
const { getSolutionInJavaScript, getSolutionInPython} = require("./utils”)
12. Tools to use consistent code style?
async_A(function(){
async_B(function(){
async_C(function(){
async_D(function(){
….
});
});
});
});
In the above coding, we are passing callback functions that make the code unreadable and not able to maintain it, which leads to changing the async logic to avoid this.
14. If Node.js is single-threaded then how it can handle concurrency?
The Node.js main loop is single-threaded and all asynchronous calls are managed by libuv library.
For example:
const crypto = require(“crypto”);
const start = Date.now();
function logHashTime() {
crypto.pbkdf2(“a”, “b”, 100000, 512, “sha512”, () => {
console.log(“Hash: “, Date.now() - start);
});
}
logHashTime();
logHashTime();
logHashTime();
logHashTime();
This gives the output:
Hash: 1213
Hash: 1225
Hash: 1212
Hash: 1222
This is because libuv creates a thread pool to manage such concurrency.
15. Explain the use of async wait in node.js?
Here is how you can use async-await pattern:
// this code is to retry with exponential backoff
function wait (timeout) {
return new Promise((resolve) => {
setTimeout(() => {
resolve()
}, timeout);
});
}
async function requestWithRetry (url) {
const MAX_RETRIES = 10;
for (let i = 0; i <= MAX_RETRIES; i++) {
try {
return await request(url);
} catch (err) {
const timeout = Math.pow(2, i);
console.log(‘Waiting’, timeout, ‘ms’);
await wait(timeout);
console.log(‘Retrying’, err.message, i);
}
}
}
Follow Simple Steps to apply in these MNC’s:
In Node.js streams are occurrences of EventEmitter that is used to work with streaming data. It is used to handle and manipulate streaming large files (such as videos, mp3, etc.) over the network.
Different types of the stream are:
19. Explain Reactor Pattern in Node.js?
20. Explain Event Emitter in Node.js?
EventEmitter is a Node.js class that involves all the objects which are fully capable of emitting events. By using an eventEmitter.on() function you can attach the named events that are released by the object. So, whenever this object emits an event the attached functions are invoked synchronously.
const EventEmitter = require(‘events’);
class MyEmitter extends EventEmitter {}
const myEmitter = new MyEmitter();
myEmitter.on(‘event’, () => {
console.log(‘an event occurred!');
});
myEmitter.emit(‘event’);
21. Explain thread pool and library that handles it in Node.js
22. What is WASI and why is it being introduced?
23. How to calculate the duration of async operations?
Performance API gives us with tools to measure performance metrics. Here are the examples of using async_hooks and perf_hooks
‘use strict’;
const async_hooks = require(‘async_hooks’);
const {
performance,
PerformanceObserver
} = require(‘perf_hooks’);
const set = new Set();
const hook = async_hooks.createHook({
init(id, type) {
if (type === ‘Timeout’) {
performance.mark(`Timeout-${id}-Init`);
set.add(id);
}
},
destroy(id) {
if (set.has(id)) {
set.delete(id);
performance.mark(`Timeout-${id}-Destroy`);
performance.measure(`Timeout-${id}`,
`Timeout-${id}-Init`,
`Timeout-${id}-Destroy`);
}
}
});
hook.enable();
const obs = new PerformanceObserver((list, observer) => {
console.log(list.getEntries()[0]);
performance.clearMarks();
observer.disconnect();
});
obs.observe({ entryTypes: [‘measure’], buffered: true });
setTimeout(() => {}, 1000);
This would give us the exact time it took to execute the callback.
24. How to calculate the performance of async operations?
Performance API gives us tools to calculate the performance metrics.
For Example:
const { PerformanceObserver, performance } = require(‘perf_hooks’);
const obs = new PerformanceObserver((items) => {
console.log(items.getEntries()[0].duration);
performance.clearMarks();
});
obs.observe({ entryTypes: [‘measure’] });
performance.measure(‘Start to Now’);
performance.mark(‘A’);
doSomeLongRunningProcess(() => {
performance.measure(‘A to Now’, ‘A’);
performance.mark(‘B’);
performance.measure(‘A to B’, ‘A’, ‘B’);
});
25. Explain callback in Node.js.
27. Where do we use Node.js most frequently?
NPM means Node Package Manager is totally responsible for handling all the packages and modules for Node.js.
Functionalities:
29. Explain the reasons why Node.js is chosen over other technologies like Java and PHP?
30. Name the database used with Node.js?
31. Name the common libraries used in Node.js?
32. Discuss the command required to import external libraries?
For importing external libraries the “require” command is used. As an example - the “var http=require (“HTTP”)” command will load the HTTP library and exported object via HTTP variable.
var http = require(‘http’);
33. Explain the meaning of event-driven programming?
34. Explain Event Loop in Node.js?
35. Explain the Express.js package?
It is basically a flexible Node.js web application framework that gives an extensive set of features to develop web and mobile-based applications.
You may also prepare:
36. Tell us the way to implement async in Node.js?
In the given code you can see that the async code requests the JavaScript engine running the code to hold the request.get() function to finish before moving to the next line for execution.
async function fun1(req, res){
let response = await request.get(‘http://localhost:3000’);
if (response.err) {console.log(‘error’);}
else { console.log(‘fetched response’);
}
37. Explain the control flow function?
38. Explain piping in Node.js?
39. Explain buffer class in Node.js?
40. Explain different types of HTTP requests?
41. Discuss first-class function in Javascript?
42. Explain Node.js and its works?
43. Tell us how Node.js overcomes the issue of blocking of I/O operations?
44. Explain the exit codes of Node.js?
It tells us how a process got terminated or the motive behind the termination.
I hope these Node.js interview questions will help you in your preparation for your upcoming Node.js technical interview. You can also prepare other coding language questions from this site.
If you are looking for a career in software development, then Jenkins is definitely worth exploring. This widely used …
In this post, we will cover a few Linux interview questions and their answers. So, let’s get started. In this …