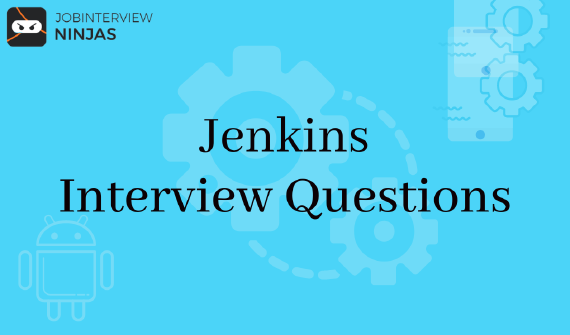
Top 40 Jenkins Interview Questions And Answers For Freshers/Experienced
If you are looking for a career in software development, then Jenkins is definitely worth exploring. This widely used …
Candidates if you are preparing for Java for your upcoming interview then you can’t miss Java Collection Interview Questions. It is an important part of Java and mostly the interviewers asked questions related to Java Collection Framework Interview Questions. So, for you here we bring the top 50 Java Collection Interview Questions and Answers which are often asked in Technical job Interviews of Developers and software engineers, etc.
About Java Collection: Basically, Java Collection is a framework that gives a structure to store and influence a set of objects. Java Collections can perform all the functions that you execute on data like searching, insertion, sorting, deletion, manipulation, etc. Java Collection represents a single unit of objects.
Also, prepare for Core Java interview questions, Networking interview questions, and other language interview questions from here.
1. Explain the framework in Java?
2. Explain the Collection framework in Java?
3. What is a Collections Class?
5. Differentiate between ArrayList and Vector in the Java collection framework.
7. Distinguish between Iterator and ListIterator
8. Distinguish between Iterator and Enumeration?
10. Define override equals() method
11. Define equals() with example
12. What are the advantages of a generic collection?
13. Tell me the way to transform ArrayList to Array and vice versa?
14. Describe the basic interfaces of the Java collections framework
15. Tell the features of Java Hashmap?
18. Tell the methods given by the Stack class
19. Explain emptySet() in the Java collections?
21. Name the collection views of a map interface
22. Tell me the advantages of the Java Collection Framework?
24. Differentiate between Set and Map?
27. Tell the two ways to delete duplicates from ArrayList?
29. Name the techniques to make collection thread-safe?
30. Define UnsupportedOperationException
31. List the collection classes that provides random element access to its elements
32. Differentiate between Queue and Deque.
33. Name Class implementing List and Set interface
34. Describe the design pattern Iterator follows.
35. Explain the peek() of the Queue interface?
36. Explain CopyOnWriteArrayList?
38. Name the techniques available in Java Queue interface
40. Explain the Java LinkedHashSet class
41. Distinguish between ArrayList and LinkedList
42. Describe the methods of iterator interface
43. Explain methods of the HashSet class?
45. Describe randomaccess interface
46. Name the collection classes that execute random access interface
47. Tell me the way to join multiple ArrayLists?
50. Name the method used to sort an array in ascending order?
1. Explain the framework in Java?
2. Explain the Collection framework in Java?
3. What is a Collections Class?
5. Differentiate between ArrayList and Vector in the Java collection framework.
7. Distinguish between Iterator and ListIterator
8. Distinguish between Iterator and Enumeration?
It is an interface utilized in Java that can expand the Queue. It gives concurrence in different queue operations like retrieval, deletion, insertion, etc.
The Queue waits to become non-empty when it is recovering any elements. BlockingQueue should not include null elements. The execution of this Queue is thread-safe.
The syntax of BlockingQueue is:
public interface BlockingQueue
10. Define override equals() method
11. Define equals() with example
Equals() confirm whether the number object is equivalent to the object, which is passed as an argument or not. The syntax of the equals() method is: public boolean equals(Object o) Example: import java.lang.Integer; public class Test { public static void main(String args[]) { Integer p = 5; Integer q = 20; Integer r =5; Short s = 5; System.out.println(p.equals(q)); System.out.println(p.equals(r)); System.out.println(p.equals(s)); } }
12. What are the advantages of a generic collection?
13. Tell me the way to transform ArrayList to Array and vice versa?
Developers can transform an Array to ArrayList by using the asList() method of the Arrays class. It is a static technique of the Arrays class that receives the List object. The syntax of the asList() method is:
Arrays.asList(item)
Java developers can transform ArrayList to the List object using syntax:
List_object.toArray(new String[List_object.size()])
14. Describe the basic interfaces of the Java collections framework
15. Tell the features of Java Hashmap?
18. Tell the methods given by the Stack class
19. Explain emptySet() in the Java collections?
Method emptySet() that brings back the blank immutable set whenever the developer tries to eliminate null elements. The set which comes back by emptySet() is serializable. The syntax is:
public static final
21. Name the collection views of a map interface
22. Tell me the advantages of the Java Collection Framework?
24. Differentiate between Set and Map?
It is a Java class that can store key-value pairs.
Know the Interview Criteria of these MNCs!!!
27. Tell the two ways to delete duplicates from ArrayList?
29. Name the techniques to make collection thread-safe?
30. Define UnsupportedOperationException
An UnsupportedOperationException is an exception or abnormality which is thrown on processes that are unsupported by the existing collection type.
For example, Programmer is creating a read-only list using “Collections.unmodifiableList(list)” and calling call(), add() or remove() method. It should clearly give UnsupportedOperationException.
31. List the collection classes that provides random element access to its elements
32. Differentiate between Queue and Deque.
33. Name Class implementing List and Set interface
Class implementing List interface: ArrayList, Vector, and LinkedList.
Class implementing Set interface: HashSet, and TreeSet.
34. Describe the design pattern Iterator follows.
35. Explain the peek() of the Queue interface?
36. Explain CopyOnWriteArrayList?
38. Name the techniques available in Java Queue interface
40. Explain the Java LinkedHashSet class
41. Distinguish between ArrayList and LinkedList
42. Describe the methods of iterator interface
43. Explain methods of the HashSet class?
45. Describe randomaccess interface
46. Name the collection classes that execute random access interface
47. Tell me the way to join multiple ArrayLists?
The list gives an addall() process that joins multiple ArrayList in Java.
For example, assume two lists 1) areaList and 2) secondAreaList. A programmer can join these ArrayLists using addall() like:
areaList.addAll(secondAreaList);
50. Name the method used to sort an array in ascending order?
If you are looking for a career in software development, then Jenkins is definitely worth exploring. This widely used …
In this post, we will cover a few Linux interview questions and their answers. So, let’s get started. In this …