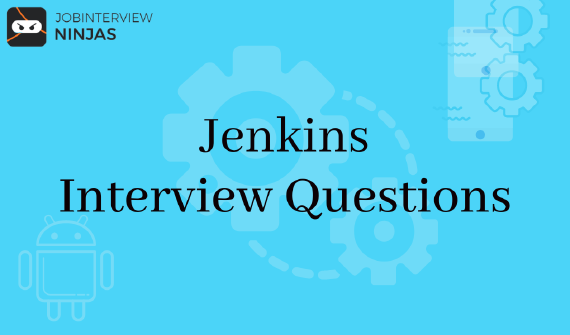
Top 40 Jenkins Interview Questions And Answers For Freshers/Experienced
If you are looking for a career in software development, then Jenkins is definitely worth exploring. This widely used …
If you are preparing for C sharp job interview then this page will be very useful to you. Here we have provided top C# interview questions to prepare. In the below section we have covered basic, intermediate, advanced, and coding C# interview questions and answers for your practice. So, prepare from the given C sharp interview questions and clear your job interview.
About C#: C sharp developed by Microsoft is an object-oriented programming language. It is basically used with the .NET framework for making sites, apps, and games.
2. Describe types of comments in C# with examples?
3. How the C# language is different from the C language?
7. Tell some use of ‘using’ statement?
9. Are we able to use the “this” command within a static method?
10. Differentiate between constants and read-only?
11. Explain interface class with example
12. Explain value types and reference types?
13. Differentiate between the System.Array.Clone() and System.Array.CopyTo()?
14. Write the code to catch an exception in C#?
15. In C# do we execute multiple catch blocks of a similar type?
16. Explain Common Language Runtime (CLR)?
17. Explain garbage collection in C#?
18. Explain different types of classes in C#?
19. Explain managed and unmanaged code?
20. Differentiate between an abstract class and an interface?
21. Differentiate between out and ref keywords?
22. Explain extension methods in C#?
23. What will happen in case inherited interfaces have method names that conflict?
24. Differentiate between a Class and a Struct?
26. Explain Boxing and Unboxing in C#?
28. Explain partial classes in C#?
29. Differentiate between late binding and early binding in C#?
30. What are the Arrays in C#?
32. Differentiate Equality Operator (==) and Equals() Method in C#?
33. Tell the various ways in which a method can be Overloaded in C#?
35. Differentiate String and StringBuilder in C#?
36. Write code to reverse a string in C# Sharp?
37. Make a code to reverse the order of the given words in C# Sharp?
38. Make a program to find whether a given string is palindrome or not in C# Sharp?
39. To find the substring from a given string write a C# program.
40. How will you find if a positive integer is prime or not? Show us through coding.
41. Differentiate between Finalize() and Dispose() methods?
42. Explain circular references?
43. Explain an object pool in .NET?
44. Name some commonly used types of exceptions in .net
45. Explain Custom Exceptions?
47. Tell the ways to inherit a class into another class in C#?
48. Tell the base class in .net from where all the classes are derived from?
49. Differentiate between method overriding and method overloading?
50. Why are you not able to specify the accessibility modifier for methods inside the interface?
2. Describe types of comments in C# with examples?
1. Single line
Example:
//This is a single line comment
2. XML Comments (///).
Eg:
/// summary;
/// Set error message for multilingual language.
/// summary
3. Multiple line (/ /)
Example:
/This is a multiple line comment
We are in line 2
Last line of comment/
3. How the C# language is different from the C language?
7. Tell some use of ‘using’ statement?
9. Are we able to use the “this” command within a static method?
10. Differentiate between constants and read-only?
11. Explain interface class with example
It is an abstract class that uses only public abstract techniques, and the methods only can declared but are not able to define. These abstract procedures need to implement in the inherited classes.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace DemoApplication
{
interface JobinterviewninjasInterface
{
void SetTutorial(int pID, string pName);
String GetTutorial();
}
class JobinterviewninjasTutorial : JobinterviewninjasInterface
{
protected int TutorialID;
protected string TutorialName;
public void SetTutorial(int pID, string pName)
{
TutorialID = pID;
TutorialName = pName;
}
public String GetTutorial()
{
return TutorialName;
}
static void Main(string[] args)
{
JobinterviewninjasTutorial pTutor = new JobinterviewninjasTutorial();
pTutor.SetTutorial(1,".Net by Jobinterviewninjas”);
Console.WriteLine(pTutor.GetTutorial());
Console.ReadKey();
}
}
}
12. Explain value types and reference types?
A value type carries a data value in its memory space. Example
int a = 30;
Reference type reserves the address of the object where the value is being kept. It acts as a pointer to another memory location.
string b = “Hello Jobinterviewninjas!!";
13. Differentiate between the System.Array.Clone() and System.Array.CopyTo()?
14. Write the code to catch an exception in C#?
We use try-catch blocks to catch an exception. The catch block needs to have a parameter of the system.Exception type.
Eg:
try {
GetAllData();
}
catch (Exception ex) {
}
15. In C# do we execute multiple catch blocks of a similar type?
16. Explain Common Language Runtime (CLR)?
17. Explain garbage collection in C#?
18. Explain different types of classes in C#?
C# has four different types of classes:
Static class: As the name itself says, the ‘static’ keyword doesn’t allow inheritance. So, you are not able to make an object for a static class.
Sample code:
static class classname
{
//static data members
//static methods
}
Partial class: As the name itself says, the ‘partial’ keyword permits its members to partially separate or share source (.cs) files.
Abstract class: Abstract classes can’t be instantiated and don’t allow you to create objects. It works on the OOPS concept of abstraction. It extracts the essential details and hides the unessential ones.
Sealed class: These classes can’t be inherited and use the keyword sealed to do the same.
sealed class Jobinterviewninjas
{
// data members
// methods
.
.
.
}
19. Explain managed and unmanaged code?
Managed code allows you to execute the code on a controlled CLR runtime environment in the .NET framework. It executes on the managed runtime atmosphere than the operating system itself. It gives services like a garbage collector, exception handling, etc.
Unmanaged code is when the code doesn’t operate on CLR, and functions outside the .NET framework. It doesn’t deliver high-level languages services and executes without them. For example C++.
20. Differentiate between an abstract class and an interface?
Example:
Abstract class:
public abstract class Shape{
public abstract void draw();
}
Interface:
public interface Paintable{
void paint();
}
21. Differentiate between out and ref keywords?
C# ref keywords pass arguments by reference and not by value. You need to mention ‘ref’ to use the ‘ref’ keyword.
void Method(ref int refArgument)
{
refArgument = refArgument + 10;
}
int number = 1;
Method(ref number);
Console.WriteLine(number);
// Output: 11
C# out keywords pass arguments within the given methods and functions. To return numerous values, the ‘out’ keyword is used to pass arguments in a method. The ref and out keywords are useful when we want to bring back a value in the similar variables that are passed as an argument.
public static string GetNextFeature(ref int id)
{
string returnText = “Next-” + id.ToString();
id += 1;
return returnText;
}
public static string GetNextFeature(out int id)
{
id = 1;
string returnText = “Next-” + id.ToString();
return returnText;
}
22. Explain extension methods in C#?
It allows us to add new methods to the current ones. The methods which will be added are static. Sometimes you want to add methods to the current class but don’t get the right to change that class then you can make a new static class using the new methods. Once the methods are declared, attach this class with the current one and notice that the methods will be added to the current one.
// C# program to illustrate the concept
// of the extension methods
using System;
namespace ExtensionMethod {
static class NewMethodClass {
// Method 4
public static void M4(this Scaler s)
{
Console.WriteLine(“Method Name: M4”);
}
// Method 5
public static void M5(this Scaler s, string str)
{
Console.WriteLine(str);
}
}
// Now we create a new class in which
// Scaler class access all the five methods
public class IB {
// Main Method
public static void Main(string[] args)
{
Scaler s = new Scaler();
s.M1();
s.M2();
s.M3();
s.M4();
s.M5(“Method Name: M5”);
}
}
}
Output:
Method Name: M1
Method Name: M2
Method Name: M3
Method Name: M4
Method Name: M5
23. What will happen in case inherited interfaces have method names that conflict?
24. Differentiate between a Class and a Struct?
Inheritance means obtaining some properties from a master class.
Here is an example of inheritance where class C can inherit properties from Class A and Class B
// C# program to illustrate
// multiple class inheritance
using System;
using System.Collections;
// Parent class 1
class Scaler {
// Providing the implementation
// of features() method
public void features()
{
// Creating ArrayList
ArrayList My_features= new ArrayList();
// Adding elements in the
// My_features ArrayList
My_features.Add(“Abstraction”);
My_features.Add(“Encapsulation”);
My_features.Add(“Inheritance”);
Console.WriteLine(“Features provided by OOPS:");
foreach(var elements in My_features)
{
Console.WriteLine(elements);
}
}
}
// Parent class 2
class Scaler2 :Scaler{
// Providing the implementation
// of courses() method
public void languages()
{
// Creating ArrayList
ArrayList My_features = new ArrayList();
// Adding elements in the
// My_features ArrayList
My_features.Add(“C++”);
My_features.Add(“C#");
My_features.Add(“JScript”);
Console.WriteLine("\nLanguages that use OOPS concepts:");
foreach(var elements in My_features)
{
Console.WriteLine(elements);
}
}
}
// Child class
class ScalertoScaler : Scaler2 {
}
public class Scaler1 {
// Main method
static public void Main()
{
// Creating object of ScalertoScaler class
ScalertoScaler obj = new ScalertoScaler();
obj.features();
obj.languages();
}
}
Also, the C# language doesn’t support multiple inheritances. Rather, you can use interfaces.
26. Explain Boxing and Unboxing in C#?
Boxing: Boxing changes value type (char, int, etc.) to reference type (object) that makes an implicit conversion method using object value.
Example:
int num = 23; // 23 will assigned to num
Object Obj = num; // Boxing
Unboxing: Unboxing changes reference type (object) to value type (char, int, etc.) by using an explicit conversion method that is opposite of it.
Example:
int num = 23; // value type is int and assigned value 23
Object Obj = num; // Boxing
int i = (int)Obj;// Unboxing
28. Explain partial classes in C#?
Partial classes apply the functionality of one class into several files. These multiple files are collected into one at the time of compilation. By using the partial keyword, a partial class is made.
public partial Clas_name
{
// code
}
You can easily divide the functionalities of procedures, interfaces, or structures into different multiple files.
29. Differentiate between late binding and early binding in C#?
Early Binding: When the binding function occurs during the compile-time, it is named early binding. It analyzes and inspects the methods and properties of static objects. It decreases the number of run-time errors substantially and it runs pretty quickly.
Late Binding: When the binding occurs at runtime, it is named late binding. Late binding occurs when the objects are dynamic at run-time. It executes slowly as it examines through during run-time.
30. What are the Arrays in C#?
When a group of the same elements is combined together under one name then the arrays are formed. The memory allotments for the elements of the array occur dynamically.
A few pointers for arrays in C#:
Syntax: < Data Type > [ ] < Name_Array >
Indexers are also known as a smart array that permits access to a member variable. Indexers permit member variables to use the features of an array. By using the Indexer keyword Indexers are made and they are not static members.
For example:
<return type> this[
{
get{
// return the value from the specified index of an internal collection
}
set{
// set values at the specified index in an internal collection
}
}
32. Differentiate Equality Operator (==) and Equals() Method in C#?
Though Equality Operator (==) and Equals() Method, both compare two objects by value, but still used differently.
For ex.:
int x = 10;
int y = 10;
Console.WriteLine( x == y);
Console.WriteLine(x.Equals(y));
Output:
True
True
33. Tell the various ways in which a method can be Overloaded in C#?
Overloading represents when a method has a similar name but holds different values to use in a separate context. The main() method cannot be overloaded other than that overloading is done in C# some of the methods are.
For example.
public class Area {
public double area(double x) {
double area = x * x;
return area;
}
public double area(double a, double b) {
double area = a * b;
return area;
}
}
During runtime, reflection abstracts metadata from the datatypes.
In the .NET framework to add reflection just use System.Refelction namespace in the programming code to retrieve the type which can be anything from the following:
35. Differentiate String and StringBuilder in C#?
The foremost difference between both of them is String objects are immutable whereas StringBuilder makes a mutable string of characters. StringBuilder does the modifications to the existing object. StringBuilder streamlines the entire procedure of constructing changes to the prevailing string object. As we know String class is immutable, it is costlier to create a new object every time we need to make a change. So, the StringBuilder class comes into the picture which can be evoked using the System.Text namespace.
In case, a string object will not change throughout the entire program, then use String class or else StringBuilder.
For ex:
string s = string.Empty;
for (i = 0; i < 1000; i++)
{
s += i.ToString() + " “;
}
Here, you’ll need to create 2001 objects out of which 2000 will be of no use.
The same can be applied using StringBuilder:
StringBuilder sb = new StringBuilder();
for (i = 0; i < 1000; i++)
{
sb.Append(i); sb.Append(’ ‘);
}
By using StringBuilder here, you also de-stress the memory allocator.
36. Write code to reverse a string in C# Sharp?
internal static void ReverseString(string str)
{
char[] charArray = str.ToCharArray();
for (int i = 0, j = str.Length - 1; i < j; i++, j–)
{
charArray[i] = str[j];
charArray[j] = str[i];
}
string reversedstring = new string(charArray);
Console.WriteLine(reversedstring);
37. Make a code to reverse the order of the given words in C# Sharp?
internal static void ReverseWordOrder(string str)
{
int i;
StringBuilder reverseSentence = new StringBuilder();
int Start = str.Length - 1;
int End = str.Length - 1;
while (Start > 0)
{
if (str[Start] == ' ‘)
{
i = Start + 1;
while (i <= End)
{
reverseSentence.Append(str[i]);
i++;
}
reverseSentence.Append(’ ‘);
End = Start - 1;
}
Start–;
}
for (i = 0; i <= End; i++)
{
reverseSentence.Append(str[i]);
}
Console.WriteLine(reverseSentence.ToString());
}
38. Make a program to find whether a given string is palindrome or not in C# Sharp?
internal static void chkPalindrome(string str)
{
bool flag = false;
for (int i = 0, j = str.Length - 1; i < str.Length / 2; i++, j–)
{
if (str[i] != str[j])
{
flag = false;
break;
}
else
flag = true;
}
if (flag)
{
Console.WriteLine(“Palindrome”);
}
else
Console.WriteLine(“Not Palindrome”);
Output:
Input: Key Output: Not Palindrome
Input: step on no pets Output: Palindrome
39. To find the substring from a given string write a C# program.
internal static void findallsubstring(string str)
{
for (int i = 0; i < str.Length; ++i)
{
StringBuilder subString = new StringBuilder(str.Length - i);
for (int j = i; j < str.Length; ++j)
{
subString.Append(str[j]);
Console.Write(subString + " “);
}
}
}
40. How will you find if a positive integer is prime or not? Show us through coding.
static void Main(string[] args)
{
if (FindPrime(47))
{
Console.WriteLine(“Prime”);
}
else
{
Console.WriteLine(“Not Prime”);
}
Console.ReadLine();
}
internal static bool FindPrime(int number)
{
if (number == 1) return false;
if (number == 2) return true;
if (number % 2 == 0) return false;
var squareRoot = (int)Math.Floor(Math.Sqrt(number));
for (int i = 3; i <= squareRoot; i += 2)
{
if (number % i == 0) return false;
}
return true;
}
41. Differentiate between Finalize() and Dispose() methods?
42. Explain circular references?
43. Explain an object pool in .NET?
44. Name some commonly used types of exceptions in .net
45. Explain Custom Exceptions?
47. Tell the ways to inherit a class into another class in C#?
A Colon can be used as an inheritance operator in C#. You just need to place a colon and then the class name.
public class DerivedClass : BaseClass
48. Tell the base class in .net from where all the classes are derived from?
49. Differentiate between method overriding and method overloading?
50. Why are you not able to specify the accessibility modifier for methods inside the interface?
Other than C# interview questions for experienced if you want to prepare for more languages then follow the below-given links or explore this website.
Want to prepare for these languages:
If you are looking for a career in software development, then Jenkins is definitely worth exploring. This widely used …
In this post, we will cover a few Linux interview questions and their answers. So, let’s get started. In this …